Previous Topic , In this tutorial we are going to learn How to Write First Cypress Test Case and execute on different browser.
How to Write First Cypress Test Case?
Whys and how to write test in Cypress? As Cypress build on java script extension. So for working on JavaScript test framework, we have follow the standards of JavaScript framework (like jasmine or mocha). They are famous java script based framework available in market as test framework, we need to follow any one of testing framework standards to write cypress testing.
Cypress has the knowledge of browser to automate. But to make a test case or script runnable we need to inject any of the cypress test framework to any of the provided java script framework, same as TestNG and junit in selenium. Cypress is recommending to use Mocha testing framework because of which Mocha comes within cypress package. So as we download cypress, Cypress automatically bundles Mocha framework.
Understanding MOCHA Structure
Before Writing First Cypress test case, lets first understand MOCHA structure. Below is the MOCHA structure
//Code Structure
describe('My First Test Suite', function(){
it('My First Test Case', function(){
})
})
When we say describe block its test suite, it nothing but test suite, there can be multiple test cases in a test suite in Mocha and it block is treated as test case. Here we have one describe block name ‘My First Test Suite’ inside this test suite we have it block which is test case and whatever we define inside the it block it is treated as a test.
In other words all the test is wrapped inside it block and all the it block is wrapped inside describe block . Both the framework MOCHA and Jasmin have same way of writing test cases.
Steps to create first test case in Cypress
Lets consider below scenario for automation.
1) Open the URL -> https://www.demoblaze.com/index.html
2) Click to SignUp on top
3) Type Username
4) Type Password
5) Click to SignUp button
Lets Automate the above scenario:
1) Click to New file button and create a spec file name as FirstTest.js as mention on screenshot below.
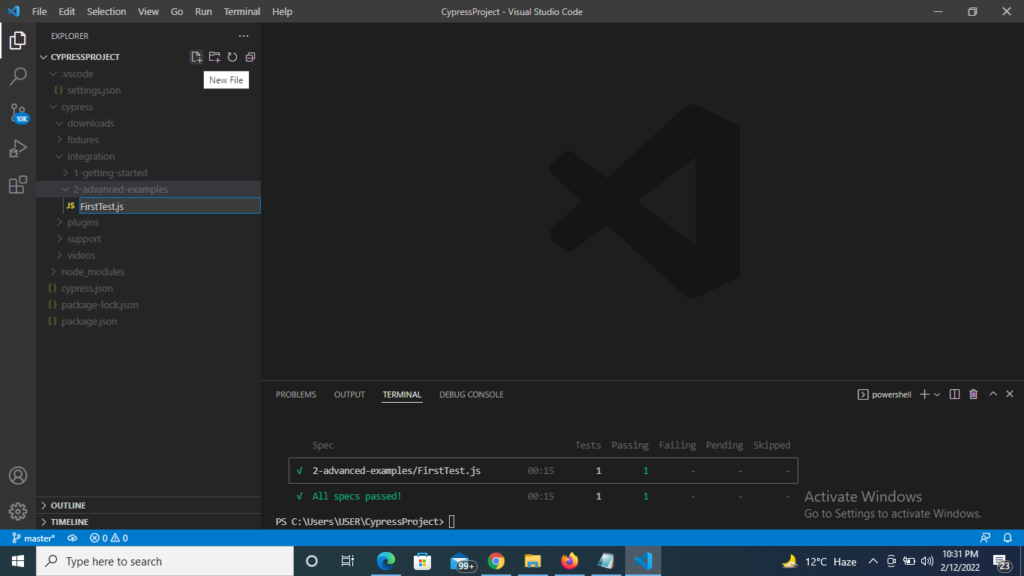
2) Copy and Paste the below code inside this FirstTest.js file.
///<reference types="cypress" />
describe('My First Test Suite', function(){
it('My First Test Case', function(){
cy.visit("https://www.demoblaze.com/index.html")
cy.wait(3000)
cy.get("#signin2").click()
cy.wait(2000)
cy.get("#sign-username").type("code2test")
cy.get("#sign-password").type("Password")
cy.get("button[onclick='register()']").click()
})
})
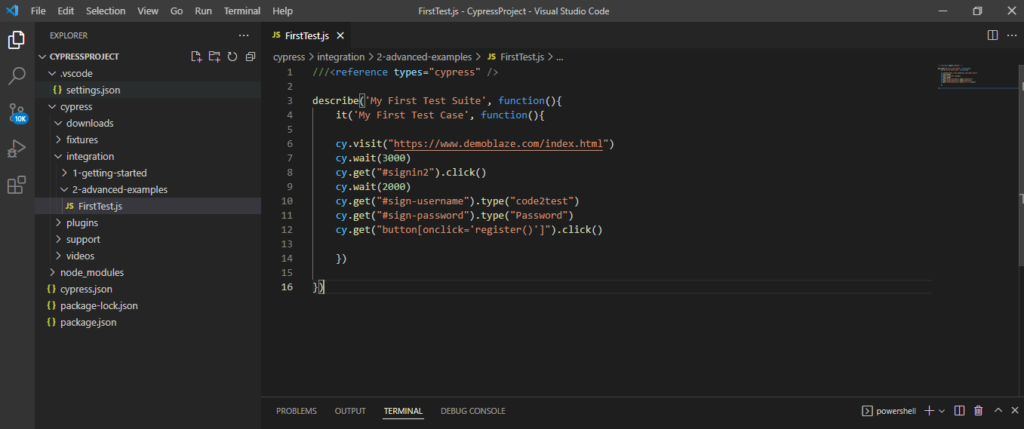
3) Now click to Terminal on the top and open a new terminal ( Terminal-> New Terminal).
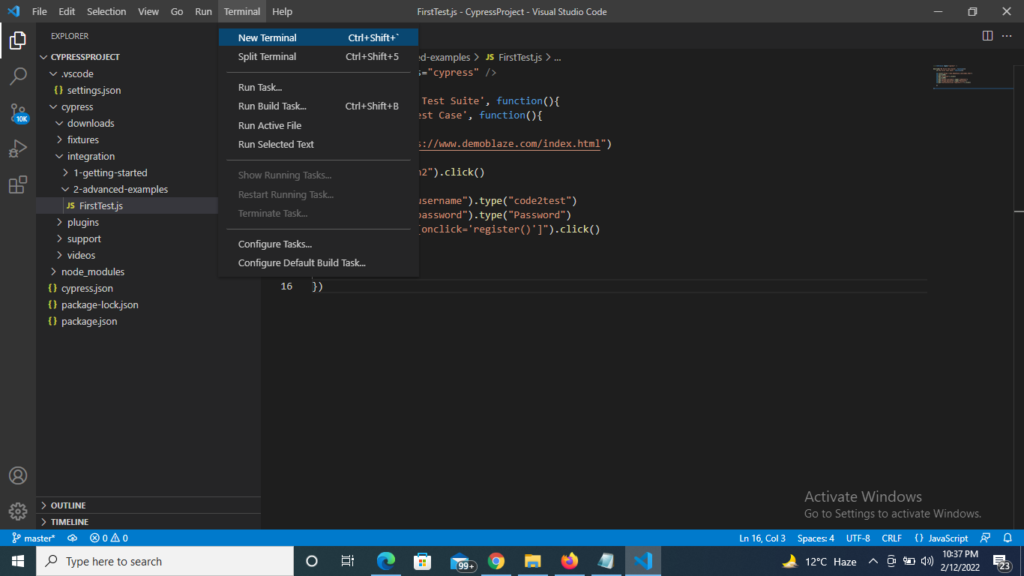
4) You will see a new terminal gets opened on the bottom of your script with different window.
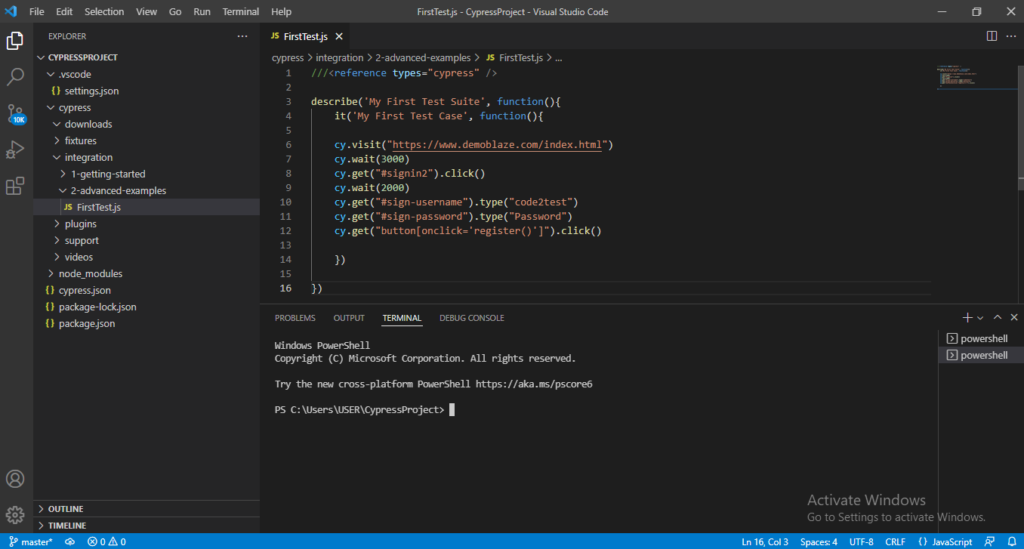
5) Now the next step is to open Cypress Test Runner by hitting below command on terminal. As this command will open the Cypress Test Runner window
node_modules\.bin\cypress open
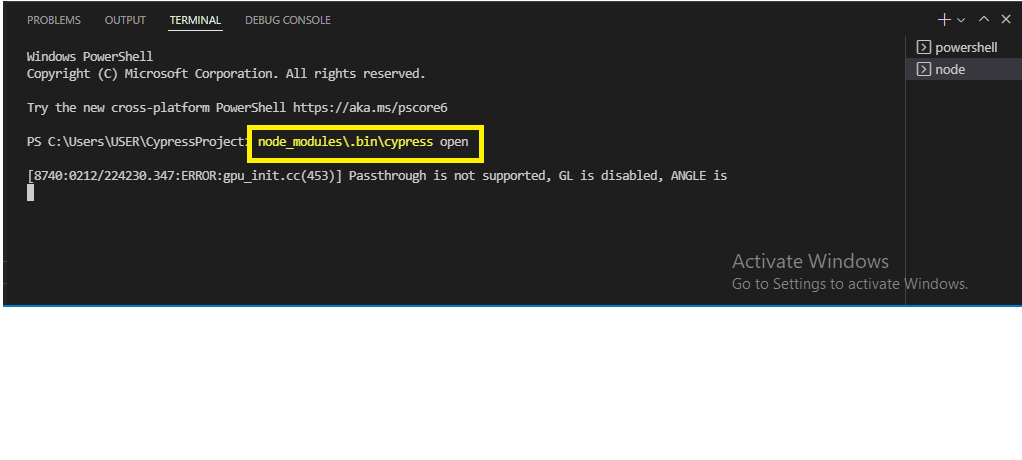
6) We see that our spec file (FirstTest.js) is visible in Cypress Test Runner window.
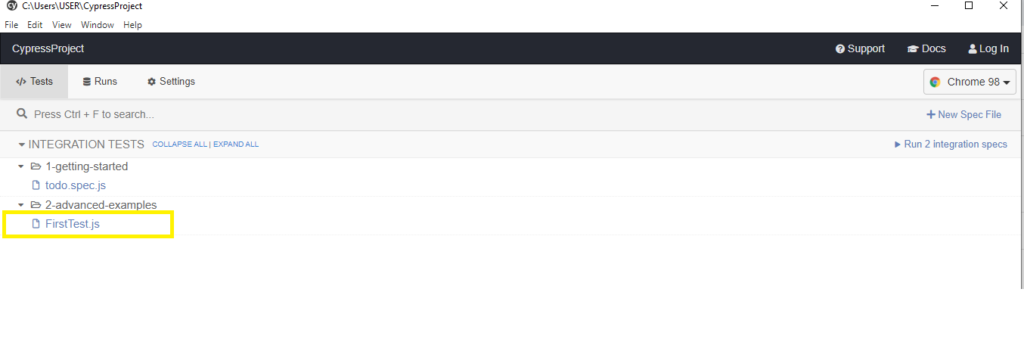
7) Now select the browser from top right list box with 3 options (Chrome, Firefox, Edge, Electron)
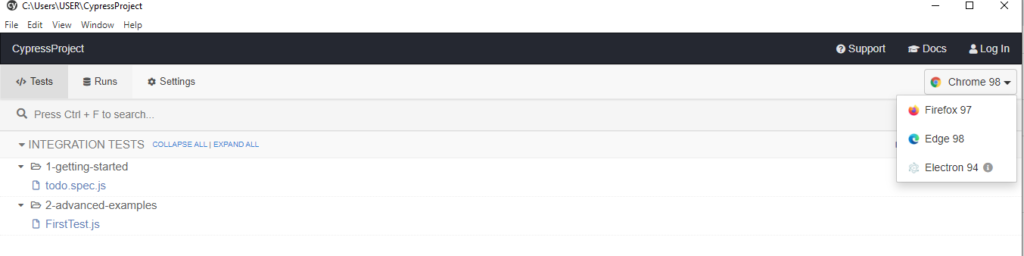
8) Suppose we selected Chrome, now double click to FirstTest.js file and execution gets start.
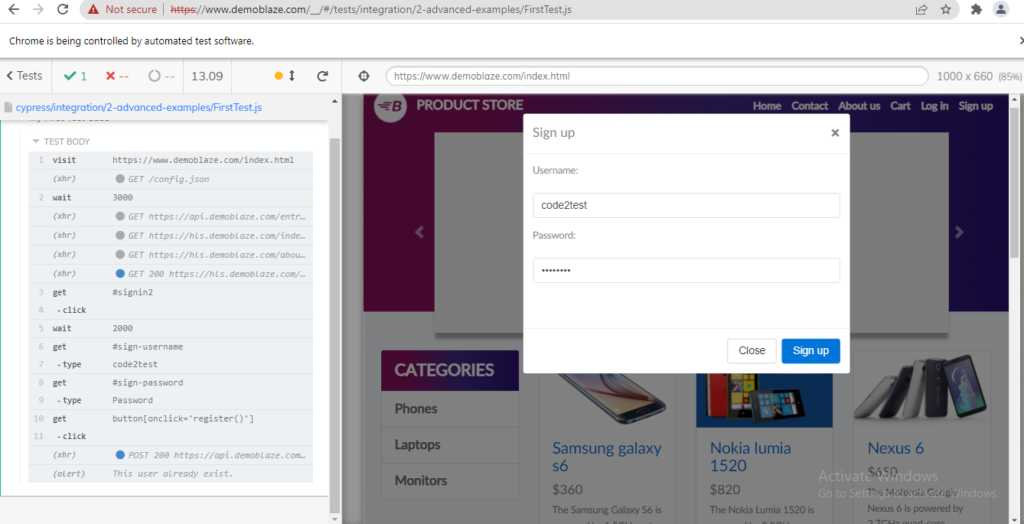
How to Run test execution through Command line or Terminal?
Below are the commands for running execution through terminal or without opening Cypress Test Runner
1) For running specific test file through terminal on default browser (Electron), hit the below command.
node_modules\.bin\cypress run --spec "cypress/integration/2-advanced-examples/FirstTest.js"
Note: This command with run the “FirstTest.js” file only and execution will perform in default (Electron) browser in headless mode (means no browser is visible to you).
2) For Running a complete test (all test files available in example folder), hit below command.
node_modules\.bin\cypress run
3) For Running specific test on headed Brower (Browser is visible to screen). Hit below command.
node_modules\.bin\cypress run --headed --spec "cypress/integration/2-advanced-examples/FirstTest.js"
Note: If we don’t specify browser it will run on default browser Electron.
4) For Running on different browser as per requirement. Hit below command.
node_modules\.bin\cypress run --browser chrome --headed --spec "cypress/integration/2-advanced-examples/FirstTest.js"
Note: On hitting above command the execution will perform in chrome browser as mentioned on code. To Run on (edge , firefox) specify or replace the browser name.
For example in firefox we use command -> node_modules.bin\cypress run –browser firefox –headed –spec “cypress/integration/2-advanced-examples/FirstTest.js”