In previous tutorial, we have learned how to parse Json Response body . In this tutorial we will discuss the concept of Serialization with Rest Assured using POJO classes
How to perform Serialization with Rest Assured?
Serialization is a process or mechanism of transforming the object state in the bit stream. In context of rest assured serialization is a process of converting the object state in the request body or payload.
As in this tutorial we are using java language to make things more simpler, In serialization we create java object from the POJO (plain old java object) class where all the attributes of the request is been defined with in a class as per the required payload structure and passed this object as a request with in the body keyword as a parameter in rest assured.
Let consider a payload as an example for which POJO class is to be created.
{
“location”: {
“lat”: -50.383494,
“lng”: 44.427362
},
“accuracy”: 70,
“name”: “Saket Terminal”,
“phone_number”: “(+91) 854 908 5667”,
“address”: “new collectorate office”,
“types”: [
“Belt teminal”,
“Commercial shop”
],
“website”: “https://code2test.com”,
“language”: “French-IN”
}
Let open this payload inside the jsoneditor, it will display as below.
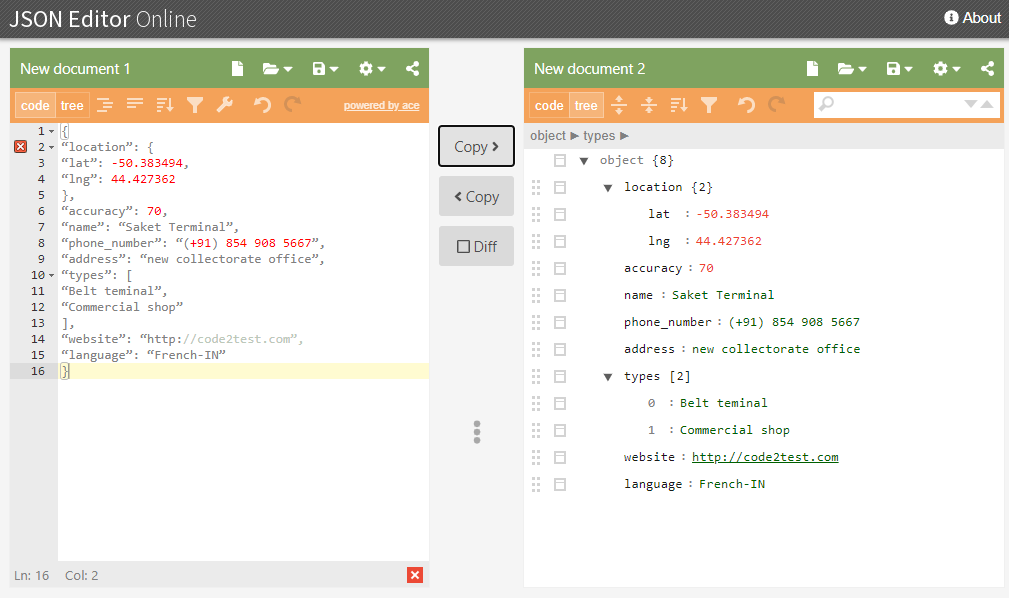
Creating POJO class (AddPlace.java) with getter and setter objects for the all the attributes present on the above JSon.
package com.restAssured;
import java.util.List;
public class AddPlace {
private int accuracy=0;
private String name=null;
private String phone_number=null;
private String address=null;
private String website=null;
private String language=null;
private Location location=null;
private List<String> types=null;
public Location getLocation() {
return location;
}
public void setLocation(Location location) {
this.location = location;
}
public int getAccuracy() {
return accuracy;
}
public void setAccuracy(int accuracy) {
this.accuracy = accuracy;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone_number() {
return phone_number;
}
public void setPhone_number(String phone_number) {
this.phone_number = phone_number;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public List<String> getTypes() {
return types;
}
public void setTypes(List<String> types) {
this.types = types;
}
public String getWebsite() {
return website;
}
public void setWebsite(String website) {
this.website = website;
}
public String getLanguage() {
return language;
}
public void setLanguage(String language) {
this.language = language;
}
}
As we need to create other POJO class (Location.java) for location attribute, as its again a json (nested JSon).
package com.restAssured;
public class Location {
public double lat;
public double lng;
public double getLat() {
return lat;
}
public void setLat(double lat) {
this.lat = lat;
}
public double getLng() {
return lng;
}
public void setLng(double lng) {
this.lng = lng;
}
}
Now create a final POJO class (RestServiceDemo.java) which provides all the values to the setter methods and finally call rest assured keywords along with methods and pass the object inside body keyword and run the script.
package com.restAssured;
import io.restassured.RestAssured;
import io.restassured.path.json.JsonPath;
import io.restassured.response.Response;
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
import java.util.ArrayList;
public class RestServiceDemo {
public static void main(String[] args) {
RestAssured.baseURI ="https://rahulshettyacademy.com";
RestAssured.useRelaxedHTTPSValidation();
AddPlace pojo= new AddPlace();
pojo.setAccuracy(70);
pojo.setAddress("Saket Terminal");
pojo.setLanguage("UnitedKingdon-IN");
pojo.setPhone_number("(+91) 8567523457");
pojo.setWebsite("https://code2test.com");
pojo.setName("new collectorate office");
ArrayList<String> list=new ArrayList<String>();
list.add("Belt Number");
list.add("Local Number");
pojo.setTypes(list);
Location loc=new Location();
loc.setLat(-34.4567);
loc.setLng(56.2453);
pojo.setLocation(loc);
Response response=given().queryParam("key","qaclick123")
.body(pojo)
.when().post("maps/api/place/add/json")
.then().log().all().assertThat().assertThat().statusCode(200).extract().response();
System.out.println("Get the response"+response.toString());
}
}
After Running the code in eclipse, the output in console display as below.
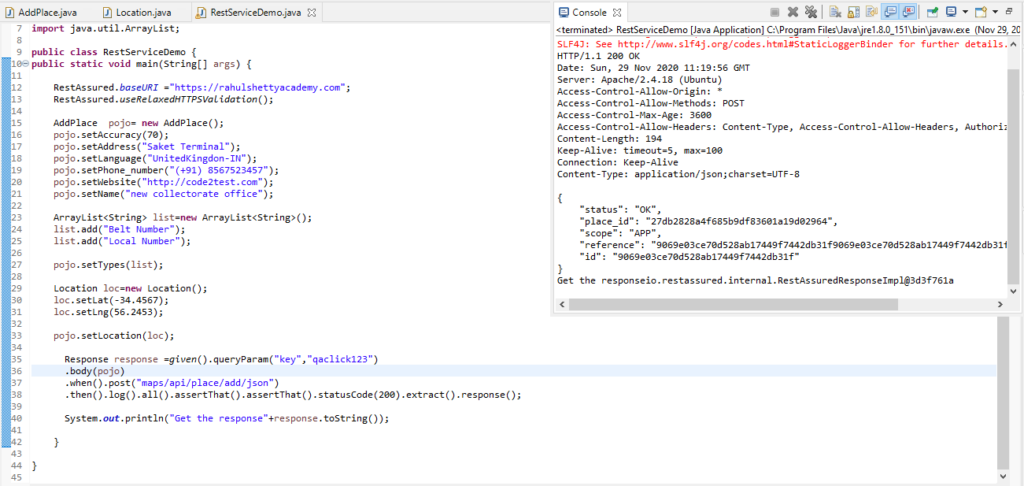
Conclusion
So in above image we see that, we received the status “OK” response or in other words successful response.
We have not passed any Json request or payload on the body keyword as parameter but the object of the POJO class. So now we are clear with the concept of serialization in our next tutorial we will understand the concept of de-serialization with rest assured.